ref函数
通过ref生成一个 引用对象(ref对象) ,定义一个响应式的数据
传统写法:打印输出的值 是 李四
,但是页面数据不变–>数据不是响应式的–>通过ref转为响应式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <template> <h2>{{name}}</h2> <h2>{{age}}</h2> <button @click="changeValue">button</button> </template>
<script> export default { setup(){ let name = "张三"; let age = 20; let changeValue=()=>{ name="李四" console.log(name); } return{ name,age,changeValue, } } } </script>
<style> </style>
|
ref转为响应式
1 2 3 4 5 6 7 8 9 10 11 12 13
| import {ref} from "vue"; export default { setup(){ let name = ref("张三"); let age = ref(20); let changeValue=()=>{ console.log(name); } return{ name,age,changeValue, } } }
|
先打印一下转化为响应式的name,是一个引用对象
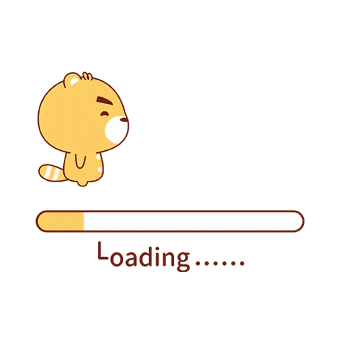
通过ref修改页面展示的数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <template> <h2>{{name}}</h2> <h2>{{age}}</h2> <button @click="changeValue">button</button> </template>
<script> import {ref} from "vue"; export default { setup(){ let name = ref("张三"); let age = ref(20); let changeValue=()=>{ name.value = "李四"; age.value = 24; } return{ name,age,changeValue, } } } </script>
<style> </style>
|
setup
中通过.value
修改ref响应式
的值;在template
的{{}}
中,不用.value
,其自动带上了.value
ref修改引用数据类型
则template
中的{{}}
需要 对象.属性
;
同基本数据类型一样,要想获取到ref里面的响应式的值,需要通过 .value 获取;
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| <template> <!-- 对象.属性 --> <h2>{{job.type}}</h2> <h2>{{job.salary}}</h2> <button @click="changeValue">button</button> </template>
<script> import {ref} from "vue"; export default { setup(){ let job =ref({ type:"前端工程师", salary:"30k" }) let changeValue=()=>{ console.log(job.value); } return{ changeValue,job } } } </script>
<style> </style>
|
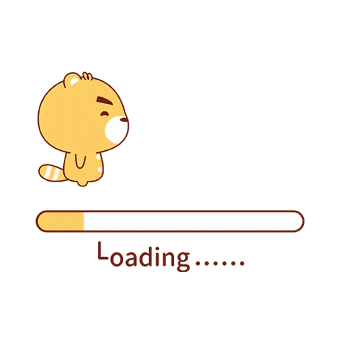
ref基本数据类型和引用数据类型
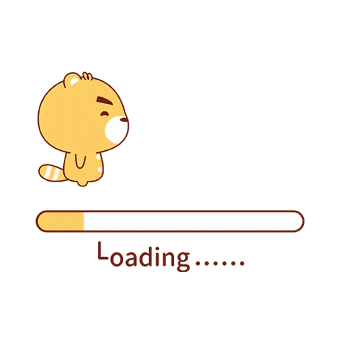
修改ref 引用数据类型的值
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| import {ref} from "vue"; export default { setup(){ let job =ref({ type:"前端工程师", salary:"30k" }) let changeValue=()=>{ job.value.type="全栈开发"; job.value.salary="60k"; } return{ changeValue,job } } }
|
reactive函数
定义一个引用数据类型的响应式数据(基本数据类型不能用它,需要用ref)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| <template> <!-- 对象.属性 --> <h2>{{job.type}}</h2> <h2>{{job.salary}}</h2> <button @click="changeValue">button</button> </template>
<script> import {reactive} from "vue"; export default { setup(){ let job =reactive({ type:"前端工程师", salary:"30k" }) let changeValue=()=>{ console.log(job); } return{ job,changeValue } } } </script>
<style> </style>
|
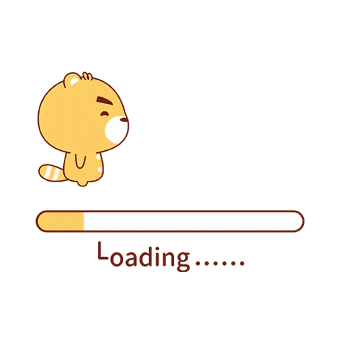
修改 reactive 创建出来 的响应式 的数据(Proxy修复了2.x,数据更新了不渲染的问题,vue.$set…)
不需要.value
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| import {reactive} from "vue"; export default { setup(){ let job =reactive({ type:"前端工程师", salary:"30k", }) let changeValue=()=>{ job.type="全栈开发"; job.salary="60k"; } return{ job,changeValue } } }
|
reactive定义的响应式对象是深层次的
;
多层嵌套的数据,也可以直接改;(实测,ref也可以直接改,毕竟ref响应式底层用的也是reactive)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| <template> <!-- 对象.属性 --> <h2>{{job.type}}</h2> <h2>{{job.salary}}</h2> <h2>{{job.a.b.c}}</h2> <button @click="changeValue">button</button> </template>
<script> import {reactive} from "vue"; export default { setup(){ let job =reactive({ type:"前端工程师", salary:"30k", a:{ b:{ c:666, } } }) let changeValue=()=>{ job.type="全栈开发"; job.salary="60k"; job.a.b.c=999; } return{ job,changeValue } } } </script>
<style> </style>
|
toRefs
创建多个ref对象
常用于对reactive对象进行解构
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| import { reactive, toRefs } from "vue"; export default { setup() { let job = reactive({ type: "前端工程师", salary: "30k", a: { b: { c: 666, }, }, }); let jieGou = toRefs(job); console.log(jieGou);
|
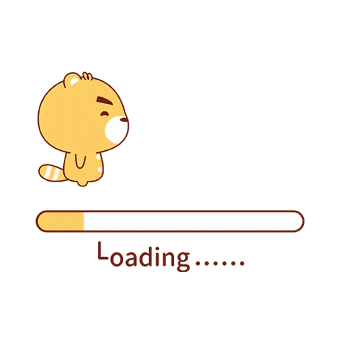
对Reactive对象进行解构
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| <template> <!-- 对象.属性 --> <!-- 因为对象结构赋值了,所以这里少写了一层 job --> <h2>{{ type }}</h2> <h2>{{ salary }}</h2> <h2>{{ a.b.c }}</h2> <button @click="changeValue">button</button> </template>
<script> import { reactive, toRefs } from "vue"; export default { setup() { let job = reactive({ type: "前端工程师", salary: "30k", a: { b: { c: 666, }, }, }); let changeValue = () => { job.type = "全栈开发"; job.salary = "60k"; job.a.b.c = 999; }; return { ...toRefs(job), changeValue, }; }, }; </script>
<style> </style>
|